Introduction
API Reference
Abstract your most important code into the processing cloud and take the burden off you dev team!
With Increase Billing you can dynamically add monthly cap, prefer low decline processors, minimize transaction costs, recover lost sales and simplify your entire card processing system. That means more time to focus on your business. You'll also get access to a team of processing specialists 24/7.
If you're a new client, view our account setup Quickstart PDF here.
The IncreaseBilling API is created around the idea of REST. The API responds to CURL requests with form-encoded request bodies, and responds with JSON-encoded responses. We also have an easy to implement PHP client library.
The PHP client can be installed using composer and is on GitLab here.
composer require rizify/increase-billingPHP <= 5.6
composer require rizify/increase-billing-legacy
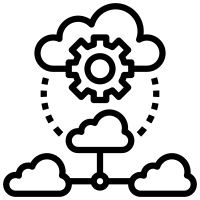
Authentication
Authentication with the IncreaseBilling API is done using an API Key and an Authorization: Bearer header to send the API Key. You can create an API Key in the Member's Area here.
If you're using the PHP library, rename src/Rizify/config_sample.ini to config.ini and add your API key and API URL there. Note the API URL is the same domain as your IncreaseBilling member's area with a sub-folder of /api/. For example: https://mysite.increasebilling.com/api/
PHP Client
require_once __DIR__ . '/vendor/autoload.php'; use Rizify\IncreaseBilling; $sm = new IncreaseBilling();
cURL
curl --location --request POST 'https://API_URL/api/sale' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \
Customer Object
The customer object represents the personally identifiable information about the customer making the transaction request. This is only used in the PHP client API to represent a customer, however, all of the request variables directly translate to the cURL requests.
Attribute | Type | Description |
---|---|---|
id | int | Reference ID # of the customer in the IncreaseBilling system |
string | Email Address of the customer | |
customer_identifier | string | Alternative to email, use this as a reference to a particular unique customer |
fname | string | First Name of the customer |
lname | string | Last Name of the customer |
phone | string | Phone # of the customer |
address1 | string | Street Address of the customer |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box |
city | string | City of Residence of the customer |
state | string | State of Residence of the customer |
country | string | Country of Residence of the customer |
postal_code | string | Postal Code of the customer |
shipping_address1 | string | Street Address of the customer |
shipping_address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box |
shipping_city | string | City of Residence of the customer |
shipping_state | string | State of Residence of the customer |
shipping_country | string | Country of Residence of the customer |
shipping_postal_code | string | Postal Code of the customer |
ip_address | string | IP Address of the customer |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([ 'fname' => 'Test', 'lname' => 'Customer', 'address1' => '3377 Test Lane', 'city' => 'New York', 'state' => 'NY', 'country' => 'US', 'postal_code' => '55555', 'email' => 'test@test.com' ]);
Order Object
The order object represents the transaction details for the customer making the request. This is only used in the PHP client API to represent an order, however, all of the request variables directly translate to the cURL requests.
Attribute | Type | Description | |
---|---|---|---|
cc_number | string | Card Number composed of 8 to 19 digits depending on the issuer | |
cc_mo | string | Card Expiration month 01 to 12 | |
cc_yr | string | Card Expiration year in the 4-digit format xxxx | |
cc_cvv | string | Card Security code | |
amount | double | Total Amount of the product or service, including shipping and taxes in format x.xx | |
price | double | Amount of the product or service, excluding shipping and taxes in format x.xx | |
tax | double | Amount of tax in format x.xx | |
shipping | double | Shipping amount in format x.xx | |
description | string | Order description, product description or any associated description of the transaction | |
vault_id | integer | IncreaseBilling customer vault ID | |
gateway_id | integer | IncreaseBilling gateway ID | |
mid_id | integer | IncreaseBilling mid ID | |
transaction_id | integer | IncreaseBilling transaction ID | |
order_id | integer | Your systems order ID, used to track transactions between your system and IncreaseBilling | |
currency | string | The transaction currency, defaults to USD. Format: ISO 4217 | |
product_id | integer | Product ID field for tracking transactions to a number based product ID. | |
product_name | string | Product Name field for tracking transactions to a string (45 character max). |
PHP Client
$sm = new IncreaseBilling(); $sm->order([ 'price' => 9.00, 'tax' => .50, 'shipping' => 0, 'cc_cvv' => 999, 'cc_number' => '4111111111111111', 'cc_yr' => '2025', 'cc_mo' => '09', 'currency' => 'USD' ]);
Response Codes
You can request this list in the API with a POST to /api/response-codes
Code | Reason | Decline Type | Retry AI | Adaptive |
---|---|---|---|---|
200 |
transaction was declined by processor |
soft | Yes | Yes |
201 |
do not honor |
soft | Yes | Yes |
202 |
insufficient funds |
soft | Yes | No |
203 |
over limit |
soft | Yes | Yes |
204 |
transaction not allowed |
hard | No | No |
220 |
incorrect payment data |
hard | No | No |
221 |
no such card issuer |
soft | Yes | Yes |
222 |
no card number on file with issuer |
hard | No | No |
223 |
expired card |
hard | No | No |
224 |
invalid expiration date |
soft | No | No |
225 |
invalid card security code |
soft | No | No |
240 |
call issuer for further information |
soft | Yes | Yes |
250 |
pick up card |
hard | No | No |
251 |
lost card |
hard | No | No |
252 |
stolen card |
hard | No | No |
253 |
fraudulent card |
hard | No | No |
260 |
declined with further instructions available (see response text) |
soft | Yes | Yes |
261 |
declined - stop all recurring payments |
hard | No | No |
262 |
declined - stop this recurring program |
hard | No | No |
263 |
declined - update cardholder data available |
soft | Yes | No |
264 |
declined - retry in a few days |
soft | Yes | No |
300 |
transaction was rejected by gateway |
hard | No | No |
400 |
transaction error returned by processor |
soft | Yes | Yes |
410 |
invalid merchant configuration |
soft | Yes | Yes |
411 |
merchant account is inactive |
soft | Yes | Yes |
420 |
communication error |
soft | Yes | Yes |
421 |
communication error with issuer |
soft | Yes | Yes |
430 |
duplicate transaction at processor |
soft | Yes | Yes |
440 |
processor format error |
soft | Yes | Yes |
441 |
invalid transaction information |
soft | Yes | Yes |
460 |
processor feature not available |
soft | Yes | Yes |
461 |
unsupported card type |
hard | No | No |
620 |
The RateOfferId did not identify a known, non-expired Reach rate offer. |
hard | No | No |
621 |
currency error |
hard | No | No |
622 |
invalid email |
hard | No | No |
623 |
invalid postal code |
hard | No | No |
624 |
invalid country |
hard | No | No |
625 |
blacklisted |
hard | No | No |
626 |
contract error |
hard | No | No |
627 |
installment error |
hard | No | No |
628 |
phone invalid |
hard | No | No |
629 |
region invalid |
hard | No | No |
630 |
birth date invalid |
hard | No | No |
631 |
national identifier invalid |
hard | No | No |
632 |
spot trade invalid |
hard | No | No |
633 |
capture invalid |
hard | No | No |
634 |
consumer invalid |
hard | No | No |
635 |
consignee invalid |
hard | No | No |
697 |
payment authentication failed |
soft | Yes | Yes |
698 |
requires action |
soft | Yes | Yes |
699 |
customer chargeback detected |
soft | No | No |
532 |
missing card token |
hard | No | No |
533 |
suspected fraud block |
hard | No | No |
534 |
merchant account closed |
hard | No | No |
993 |
no reach decline map |
soft | Yes | No |
994 |
no braintree decline map |
soft | Yes | No |
995 |
no authorize decline map |
soft | Yes | No |
996 |
no payshield decline map |
soft | Yes | No |
997 |
no cybersource decline map |
soft | Yes | No |
998 |
no stripe decline map |
soft | Yes | No |
999 |
server error |
soft | Yes | Yes |
Currency
ISO 4217 format currency codes. The default currency is USD, if you want to process using another currency, pass the ISO 4217 currency code to the API. For a list of currency codes click here. See Order Object for request information.
PHP Client
require_once __DIR__ . '/vendor/autoload.php'; use Rizify\IncreaseBilling; $sm = new IncreaseBilling(); $sm->order([ 'price' => 9.00, 'tax' => .50, 'shipping' => 0, 'cc_cvv' => 999, 'cc_number' => '4111111111111111', 'cc_yr' => '2025', 'cc_mo' => '09', 'currency' => 'USD' ]);
cURL
curl --location --request POST 'https://API_URL/api/sale' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'currency=""' \
Checkout JS
Use this checkout page script if:
- You aren't PCI Compliant
- You need to support 3DS on your checkout page
- You use a gateway type that requires device fingerprints
Not PCI Compliant? This set up will ensure card information never touches your server.
Getting Started
There are two primary features with this script, card tokenization, and transactions that require additional actions (like 3DS).
Implement one or both of the options below!
Copy-paste the script link from your member area Checkout JS menu link, it looks like this:
Option 1: Card Tokenization
1. Add tags to your checkout page which we'll use to collect the card info:
2. Configure the script tag, replacing data-tokenization-key with the key and data-url API URL found in your IncreaseBilling site.
3. Add id="payToken" to your checkout button
4. When you POST the form, there will be a POST field 'payment_token' which you can use to process a sale with (one time use), rather than the raw Card info.
Option 2: 3DS and Device Fingerprints
1. Add a hidden input with ID "device_fingerprint" (also accessible in JS with window.gip_device_fingerprint), post this to the API for the sale request if not empty
2. If you get a decline with a message of "requires_action" (e.g. transaction requires 3DS):
- Step 1: Extract 'meta' from Transaction Result
- Stringify the meta property.
- Pass the
"meta"
property to the JavaScript functionrequiredAction((string) metaProperty, (function) callback)
.
- Step 2: From the callback function pass the "result" to your server and send to Increase Billing's "/api/requires-action-confirmation"
route to confirm the result of the user interaction (3DS modal results)
- The response is a standard transaction result which you then process like normal, either a success response or decline with message.
- If the transaction was declined show the customer the message (Insufficient Funds, etc).
- If successful, redirect customer to the next destination.
Javascript Callback example:
Server-side PHP Example:
Advanced Script Options
data-bootstrap: If you're using Bootstrap we'll add form-control classes to the inputs
data-fail-message: Customize a server side failure message, these are rare, but just in case you get an empty response from the API, we'll show this error message.
data-year-type: Use "select" for a dropdown select box, or input for a text input.
data-month-type: Use "select" for a dropdown select box, or input for a text input.
Test Cards
Card Scheme | Card Number (PAN) |
---|---|
Amex | 371111111111114 |
Diners | 3001111111111116 |
Discover | 6011111111111117 |
JCB | 35281111111111119 |
Maestro | 56410411111111113 |
Mastercard (Credit) | 5411111111111115 |
Mastercard (Debit) | 5511111111111114 |
Visa (Credit) | 4532111111111112 |
Visa (Debit) | 4751271111111118 |
Test Cards To Simulate AVS
Card Scheme | Card Number (PAN) | CSC | Address | Postcode |
---|---|---|---|---|
Amex | 376100000000004 | 3761 | 4 Amex Street, Southampton | SO31 6XY |
Amex | 341111597241002 | 1111 | 27 Broadway, New York | 10004-1601 |
Diners | 36555500001111 | Diners Club UK Ltd, 53 Diners Road, Salford | M5 3BH | |
Discover | 6011000991300009 | 444 | 16 5TH ST SE, Washington DC | 20003-1120 |
JCB | 3561000000000005 | 356 | 5 JCB Street, Hereford | HR3 5TR |
JCB | 3566000020000410 | Flat 10, 47 Park Street, London | W1K 7EB | |
Maestro | 6761000000000006 | 676 | 6 Maestro Street, Exeter | EX16 7EF |
Maestro | 6333000023456788 | 888 | 1 Bd Victor, Paris, France | 75015 |
Mastercard | 5123450000000008 | 512 | 56 Gloucester Road, Glasgow | GL1 2US |
Mastercard | 5301250070000191 | 999 | 73 Whiteladies Road, Clifton, Bristol | BS8 2NT |
Mastercard | 5413330089600010 | 541 | 107 Central Park West, New York | 10023 |
Mastercard | 5413330089099049 | 541 | 1116 Amsterdam Ave, New York | 10027 |
Mastercard | 5761000000000008 | 576 | 8 Mastercard Street, Highbridge | TA6 4GA |
Visa | 4012000033330026 | 123 | 123 Fake Street, Sprinfield | SF1 234 |
Visa | 4123450131003312 | 412 | 782 Northampton Lane, Hull | HL8 2UA |
Visa | 4111111111111111 | 28 Bishopgate Street, Sedgeford | PE36 4AW | |
Visa | 4539791001730106 | 222 | 34 Broadway, New York | 10004-1608 |
Visa | 4761000000000001 | 476 | 1 Visa Street, Crewe | CW4 7NT |
Visa | 4761739001010010 | 476 | 84 Hudson St, New York | 10013 |
Visa | 4761739001012222 | 476 | 315 W 36th St, New York | 10018 |
* For expiry date, please choose any date in the future
** For CSC/CVV, please use the first 3 digits of the card number (PAN).
How do you trigger errors in sandbox mode?
- To cause a declined message, pass an amount less than 1.00.
- To trigger a fatal error message, pass an invalid card number.
- To simulate an AVS match, pass 888 in the address1 field, 77777 for zip.
- To simulate a CVV match, pass 999 in the cvv field.
3DS
If your transaction supports 3DS Authentication pass these variables to any transaction route where it is applicable and we'll save the transaction along with the 3DS values, and pass the 3DS values to your gateway. Please contact support@increasebilling.com if you have questions!
Attribute | Type | Required | Description |
---|---|---|---|
three_ds_authentication_value | string | * | Cardholder Authentication Verification Value for EMV 3DS. Required on success only. *Alternatively use three_ds_authentication_value (these are functionally the same) |
three_ds_version | string | EMV 3DS version 2.1.0, 2.2.0, 2.3.1 | |
three_ds_status | string | Y, A, C, N, U, R, I status codes. Check 3DS documentation for significance. | |
eci | string | 3DS authentication attempt value | |
xid | string | The transaction identifier (XID) is a unique tracking number set by the merchant for 3DS 1.0 | |
cavv | string | * | Cardholder Authentication Verification Value for 3DS 1.0. Required on success only. *Alternatively use three_ds_authentication_value (these are functionally the same) |
acs_trans_id | string | ACS Transaction ID | |
ds_trans_id | string | Directory Server Transaction ID | |
three_ds_trans_status_reason | string | 3DS Transaction Status Reason. Check 3DS documentation for significance. | |
cardholder_auth | string | A string describing if a customer was successfully verified or attempted. Examples: verified, attempted |
What is EMV 3-D Secure (EMV 3DS)?
Fighting payment fraud and checkout friction is key to businesses delivering a safe and convenient digital shopping experience for their customers. Payment card issuers and merchants use EMV 3DS to seamlessly authenticate consumers and safeguard against card-not-present (CNP) fraud.
EMV 3DS enables the exchange of data, or messages, between the merchant and the issuer to authenticate the consumer and approve the transaction. The data includes information about the transaction, payment method and device. Using this data, issuers can identify and prevent fraudulent card transactions quickly and accurately, without adding unnecessary friction to the payment process that often leads to abandoned purchases.
The EMV 3DS Specifications provide a common set of requirements that product providers can use to integrate this technology into their solutions to support seamless and secure e-commerce payments.
Authorize
POST /api/authorization
Authorize a charge that can be 'captured' (see Capture request) later. Save the transaction_id and gateway_id for the capture request.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
phone | string | Phone # of the customer | |
product_id | string | Product ID field for tracking transactions to a number based product ID. (50 character max) | |
product_name | string | Product Name field for tracking transactions to a string (100 character max). | |
product_category | string | Product Category field for tracking transactions to a string (100 character max). | |
payment_token | string | * | Payment token from Checkout JS, required if no card data is included with the request |
cc_number | string | * | Card Number composed of 8 to 19 digits depending on the issuer, required if no payment_token is included with the request |
cc_mo | string | * | Card Expiration month 01 to 12, required if no payment_token is included with the request |
cc_yr | string | * | Card Expiration year in the 4-digit format xxxx, required if no payment_token is included with the request |
cc_cvv | string | Card Security code | |
amount | double | Total Amount of the product or service, including shipping and taxes in format x.xx | |
price | double | Amount of the sale transaction, excluding shipping and taxes in format x.xx | |
tax | double | Amount of tax in format x.xx | |
shipping | double | Shipping amount in format x.xx | |
gateway_group_id | integer | IncreaseBilling gateway GROUP ID to process transaction, you can configure gateway groups in the member area | |
vault_add | integer | Send "1" to create a vault_id if the transaction is successful, results in response->meta->vault_id, make sure to save response->meta->gateway_id for future charges. | |
card_add | integer | Send "1" to create a tokenized card which can be used for future billing with a Recurring request, results in response->meta->vault_id. | |
gateway_id | integer | IncreaseBilling gateway ID to process transaction | |
mid_id | integer | IncreaseBilling mid ID to process transaction in specific mid-gateway |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->authorization();
cURL
curl --location --request POST 'https://API_URL/api/authorization' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'fname=""' \ --form 'lname=""' \ --form 'email=""' \ --form 'address1=""' \ --form 'city=""' \ --form 'state=""' \ --form 'country=""' \ --form 'postal_code=""' \ --form 'gateway_id=""' \ --form 'mid=""' \ --form 'price=""' \ --form 'tax=""' \ --form 'shipping=""' \ --form 'cc_number=""' \ --form 'cc_mo=""' \ --form 'cc_yr=""' \ --form 'cc_ccv=""' \ --form 'gateway=""' \ --form 'amount=""'
Response
{ "success": 1, "message": "SUCCESS", "meta": [], "transaction": { "user_id": 1, "customer_id": 4, "gateway_id": 58, "mid_id": null, "total": "50.10", "price": "50.00", "tax": ".05", "shipping": ".05", "response": "1", "response_code": "100", "response_text": "SUCCESS", "avsresponse": "", "cvvresponse": "M", "order_id": "141655137213", "transaction_id": "7320273409", "type": "sale", "cc_number": "4xxxxxxxxxxx1111", "vault_id": "", "checkaba": "", "checkaccount": "", "authcode": "123456", "tracking_campaigns_id": "5", "sandbox": 1, "ai_used": null, "ip_address": "147.219.60.240", "bin_list_id": null, "gateway_type": "nmi", "subscription_id": null, "first_six": "411111", "last_four": "1111", "updated_at": "2022-06-13T16:20:16.000000Z", "created_at": "2022-06-13T16:20:16.000000Z", "id": 258106 }, "customer": { "id": 4, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-11-05T00:17:04.000000Z", "updated_at": "2022-06-13T15:49:07.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288" } }
Capture
POST /api/capture
Capture an authorized transaction to settle the payment, using the transaction_id and gateway_id from the Authorization request. Note this creates a sale transaction if successful.
Attribute | Type | Required | Description |
---|---|---|---|
gateway_id | integer | IncreaseBilling gateway ID from the authorization request | |
transaction_id | integer | IncreaseBilling transaction ID from the authorization request to capture |
PHP Client
$sm = new IncreaseBilling(); $sm->order([REQUIRED FIELDS]); $result = $sm->capture();
cURL
curl --location --request POST 'https://API_URL/api/capture' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'transaction_id=""' \ --form 'gateway_id=""' \ --form 'amount=""'
Response
{ "success": 1, "message": "SUCCESS", "meta": [], "transaction": { "user_id": 1, "customer_id": 1, "gateway_id": 3, "gateway_type": "nmi", "mid_id": null, "sandbox": 1, "order_id": "", "ai_used": null, "tracking_campaigns_id": null, "product_name": null, "type": "sale", "total": "1.50", "price": null, "shipping": null, "tax": null, "response": 1, "response_code": 100, "response_text": "SUCCESS", "authcode": 123456, "transaction_id": "7321149525", "avsresponse": "N", "cvvresponse": "", "checkaccount": "", "vault_id": "865723865", "checkaba": "", "cc_number": "4xxxxxxxxxxx1111", "first_six": null, "last_four": null, "bin_list_id": null, "ip_address": "147.219.60.240", "linked_transactions_id": 258112, "subscription_id": null, "updated_at": "2022-06-13T21:06:42.000000Z", "created_at": "2022-06-13T21:06:42.000000Z", "id": 258113 }, "customer": { "id": 1, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-06-08T22:40:17.000000Z", "updated_at": "2022-06-13T16:59:09.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288" } }
Create a Tokenized Card For Future Billing
POST /api/card-add
Add a customer and tokenize their card information, which can be used to process sale charges without storing the card on your server.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
phone | string | Phone # of the customer | |
cc_number | string | Card Number composed of 8 to 19 digits depending on the issuer | |
cc_mo | string | Card Expiration month 01 to 12 | |
cc_yr | string | Card Expiration year in the 4-digit format xxxx | |
cc_cvv | string | Card Security code |
cURL
curl --location --request POST 'https://API_URL/api/card-add' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'fname=""' \ --form 'lname=""' \ --form 'email=""' \ --form 'address1=""' \ --form 'city=""' \ --form 'state=""' \ --form 'country="US"' \ --form 'postal_code=""' \ --form 'shipping=""' \ --form 'cc_number=""' \ --form 'cc_mo=""' \ --form 'cc_yr=""' \ --form 'cc_ccv=""' \ --form 'gateway_id=""' \
Response
{ "success": 1, "message": "Customer Added", meta": { "vault_id": "17672-internal", "gateway_id": x }, "transaction": { "user_id": 1, "customer_id": x, "gateway_id": x, "mid_id": null, "total": null, "price": null, "tax": null, "shipping": null, "response": "1", "response_code": "100", "response_text": "Customer Added", "avsresponse": "", "cvvresponse": "", "order_id": "1219991680279618", "transaction_id": "", "type": "vault_add", "vault_id": "x", "checkaba": null, "checkaccount": null, "authcode": "", "tracking_campaigns_id": null, "sandbox": null, "ai_used": null, "ip_address": "x", "bin_list_id": 83983, "gateway_type": "x", "subscription_id": null, "cc_number": null, "first_six": "411111", "last_four": "1111", "card_type": "VISA", "id": 122296 } }
Chargeback
POST /api/chargeback
Flag a transaction ID as chargedback
Attribute | Type | Required | Description |
---|---|---|---|
transaction_id | integer | IncreaseBilling transaction ID |
PHP Client
$sm = new IncreaseBilling(); $sm->order([REQUIRED FIELDS]); $result = $sm->chargeback();
cURL
curl --location --request POST 'https://API_URL/api/chargeback' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'transaction_id=""' \
Response
{ "success": 1, "message": "created chargeback transaction for transaction_id 7385547143", "meta": [], "transaction": { "user_id": 1, "customer_id": 1, "gateway_id": 3, "gateway_type": "nmi", "mid_id": null, "sandbox": 1, "order_id": "1586331657122846", "ai_used": null, "tracking_campaigns_id": null, "product_name": null, "type": "chargeback", "total": "12.00", "price": "10.00", "shipping": "1.50", "tax": "0.50", "response": 1, "response_code": 100, "response_text": "SUCCESS", "authcode": 123456, "transaction_id": "7385547143", "avsresponse": "N", "cvvresponse": "M", "checkaccount": "", "vault_id": "", "checkaba": "", "cc_number": "4xxxxxxxxxxx1111", "first_six": "411111", "last_four": "1111", "bin_list_id": null, "ip_address": "174.247.27.102", "linked_transactions_id": 258159, "subscription_id": null, "product_id": null, "capture": null, "created_at": "2022-08-18T13:55:59.000000Z", "updated_at": "2022-08-18T13:55:59.000000Z", "id": 258211 }, "customer": { "id": 1, "user_id": 1, "email": "", "fname": null, "lname": null, "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2022-07-06T15:52:10.000000Z", "updated_at": "2022-07-06T20:08:09.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": null } }
Create Customer
POST /api/create-customer
Create a customer in the IncreaseBilling system
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
phone | string | Phone # of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
shipping_address1 | string | Street Address of the customer | |
shipping_address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
shipping_city | string | City of Residence of the customer | |
shipping_state | string | State of Residence of the customer | |
shipping_country | string | Country of Residence of the customer | |
shipping_postal_code | string | Postal Code of the customer | |
ip_address | string | IP Address of the customer |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->createCustomer();
cURL
curl --location --request POST 'https://API_URL/api/create-customer' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'transaction_id=""' \ --form 'gateway_id=""' \
Response
{ "success": 1, "message": "Customer created!", "meta": [], "transaction": null, "customer": { "id": 11, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "Madison", "state": "WI", "country": "US", "postal_code": "", "phone": null, "shipping_address1": "", "shipping_address2": "", "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2022-01-21T16:13:40.000000Z", "updated_at": "2022-01-21T16:16:08.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": 7272727 } }
Create Customer Vault
POST /api/create-customer-vault
Create a vault ID in the IncreaseBilling system and associate it with an existing customer
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
gateway_id | int | Gateway ID that the vault ID ALREADY exists in | |
vault_id | string | Vault ID that already exists in the gateway |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->createCustomerVault();
cURL
curl --location --request POST 'https://API_URL/api/create-customer-vault' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'transaction_id=""' \ --form 'gateway=""' \
Response
{ "success": 1, "message": "Customer vault created!", "meta": [], "transaction": null, "customer": { "id": 11, "user_id": 1, "email": "atesting15@thing.com", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": "", "shipping_address2": "", "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2022-01-21T16:13:40.000000Z", "updated_at": "2022-06-13T19:40:20.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": null, "vault": [ { "id": 93, "user_id": null, "customer_id": 11, "vault_id": "99999999", "gateway_id": 3, "processor_id": null, "billed": 0, "bin_list_id": null, "created_at": "2022-01-21T18:05:40.000000Z", "updated_at": "2022-01-21T18:05:40.000000Z", "card_updated_date": null } ] } }
Sale
POST /api/sale
Transaction sales are submitted and immediately flagged for settlement.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
phone | string | Phone # of the customer | |
product_id | string | Product ID field for tracking transactions to a number based product ID. (50 character max) | |
product_name | string | Product Name field for tracking transactions to a string (100 character max). | |
product_category | string | Product Category field for tracking transactions to a string (100 character max). | |
payment_token | string | * | Payment token from Checkout JS, required if no card data is included with the request |
cc_number | string | * | Card Number composed of 8 to 19 digits depending on the issuer, required if no payment_token is included with the request |
cc_mo | string | * | Card Expiration month 01 to 12, required if no payment_token is included with the request |
cc_yr | string | * | Card Expiration year in the 4-digit format xxxx, required if no payment_token is included with the request |
cc_cvv | string | Card Security code | |
price | double | Amount of the sale transaction, excluding shipping and taxes in format x.xx | |
tax | double | Amount of tax in format x.xx | |
shipping | double | Shipping amount in format x.xx | |
gateway_id | integer | IncreaseBilling gateway ID to process transaction | |
gateway_group_id | integer | IncreaseBilling gateway GROUP ID to process transaction, you can configure gateway groups in the member area | |
mid_id | integer | IncreaseBilling mid ID to process transaction in specific mid-gateway | |
vault_add | integer | Send "1" to create a vault_id if the transaction is successful, results in response->meta->vault_id, make sure to save response->meta->gateway_id for future charges. | |
card_add | integer | Send "1" to create a tokenized card which can be used for future billing with a Recurring request, results in response->meta->vault_id. | |
subscription_id | integer | On successful charge add the customer to a subscription ID (create the subscription model and retrieve its ID with the API or Increase Billing member's area). | |
3DS | Add 3DS authentication to your transactions, check documentation on left-side menu. | ||
googlepay_payment_data | string | NMI Only | The encrypted token created by the Google Pay SDK. |
applepay_payment_data | string | NMI Only | The encrypted Apple Pay payment data (payment.token.paymentData) from PassKit encoded as a hexadecimal string |
device_fingerprint | string | * Required WithReach Only | Use our checkout javascript to generate a device fingerprint which you pass with the sale request |
paypal | int | * WithReach Gateway Only | Process a sale through Paypal-Reach, also requires device_fingerprint and return_url |
return_url | string | After some action occurs that redirects a customer from a checkout page, return customer to the return_url defined here |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->validate();
cURL
curl --location --request POST 'https://API_URL/api/sale' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'fname=""' \ --form 'lname=""' \ --form 'email=""' \ --form 'address1=""' \ --form 'city=""' \ --form 'state=""' \ --form 'country=""' \ --form 'postal_code=""' \ --form 'gateway_id=""' \ --form 'mid=""' \ --form 'price=""' \ --form 'tax=""' \ --form 'shipping=""' \ --form 'cc_number=""' \ --form 'cc_mo=""' \ --form 'cc_yr=""' \ --form 'cc_ccv=""' \ --form 'gateway=""' \ --form 'amount=""'
Response
{ "success": 1, "message": "SUCCESS", "meta": [], "transaction": { "user_id": 1, "customer_id": 4, "gateway_id": 58, "mid_id": null, "total": "50.10", "price": "50.00", "tax": ".05", "shipping": ".05", "response": "1", "response_code": "100", "response_text": "SUCCESS", "avsresponse": "", "cvvresponse": "M", "order_id": "141655137213", "transaction_id": "7320273409", "type": "sale", "cc_number": "4xxxxxxxxxxx1111", "vault_id": "", "checkaba": "", "checkaccount": "", "authcode": "123456", "tracking_campaigns_id": "5", "sandbox": 1, "ai_used": null, "ip_address": "147.219.60.240", "bin_list_id": null, "gateway_type": "nmi", "subscription_id": null, "first_six": "411111", "last_four": "1111", "updated_at": "2022-06-13T16:20:16.000000Z", "created_at": "2022-06-13T16:20:16.000000Z", "id": 258106 }, "customer": { "id": 4, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-11-05T00:17:04.000000Z", "updated_at": "2022-06-13T15:49:07.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288" } }
Adaptive Payment Response
{ "success": 1, "message": "SUCCESS", "meta": { "adaptive_payment": { "original_sale": { "user_id": 1, "customer_id": 21999, "gateway_id": 60, "mid_id": null, "total": 0.05, "price": ".05", "tax": ".0", "shipping": ".0", "response": "2", "response_code": "200", "response_text": "DECLINE", "avsresponse": "N", "cvvresponse": "M", "order_id": "1219991680542444", "transaction_id": "8214868379", "type": "sale", "vault_id": null, "checkaba": null, "checkaccount": null, "authcode": "", "tracking_campaigns_id": null, "sandbox": null, "ai_used": null, "ip_address": "71.15.83.29", "bin_list_id": 83983, "gateway_type": "nmi", "subscription_id": null, "cc_number": null, "first_six": "411111", "last_four": "1111", "card_type": "VISA", "payment_token_id": null, "product_name": null, "product_id": null, "products": null, "updated_at": "2023-04-03T17:20:44.000000Z", "created_at": "2023-04-03T17:20:44.000000Z", "id": 122368 }, "validate": { "user_id": 1, "customer_id": 21999, "gateway_id": 3, "mid_id": null, "total": null, "price": null, "tax": null, "shipping": null, "response": "1", "response_code": "100", "response_text": "SUCCESS", "avsresponse": "N", "cvvresponse": "M", "order_id": "1219991680542446", "transaction_id": "8214868492", "type": "validate", "vault_id": null, "checkaba": null, "checkaccount": null, "authcode": "", "tracking_campaigns_id": null, "sandbox": null, "ai_used": 2, "ip_address": "71.15.83.29", "bin_list_id": 83983, "gateway_type": "nmi", "subscription_id": null, "cc_number": null, "first_six": "411111", "last_four": "1111", "card_type": "VISA", "payment_token_id": null, "product_name": null, "product_id": null, "products": null, "updated_at": "2023-04-03T17:20:46.000000Z", "created_at": "2023-04-03T17:20:46.000000Z", "id": 122369 } } }, "transaction": { "user_id": 1, "customer_id": 4, "gateway_id": 58, "mid_id": null, "total": "50.10", "price": "50.00", "tax": ".05", "shipping": ".05", "response": "1", "response_code": "100", "response_text": "SUCCESS", "avsresponse": "", "cvvresponse": "M", "order_id": "141655137213", "transaction_id": "7320273409", "type": "sale", "cc_number": "4xxxxxxxxxxx1111", "vault_id": "", "checkaba": "", "checkaccount": "", "authcode": "123456", "tracking_campaigns_id": "5", "sandbox": 1, "ai_used": null, "ip_address": "147.219.60.240", "bin_list_id": null, "gateway_type": "nmi", "subscription_id": null, "first_six": "411111", "last_four": "1111", "updated_at": "2022-06-13T16:20:16.000000Z", "created_at": "2022-06-13T16:20:16.000000Z", "id": 258106 }, "customer": { "id": 4, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-11-05T00:17:04.000000Z", "updated_at": "2022-06-13T15:49:07.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288" } }
Create a Recurring Customer
POST /api/vault-add
Add a customer to the "vault" along with their card information, which can be used to process sale charges without storing the card on your server.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
phone | string | Phone # of the customer | |
cc_number | string | Card Number composed of 8 to 19 digits depending on the issuer | |
cc_mo | string | Card Expiration month 01 to 12 | |
cc_yr | string | Card Expiration year in the 4-digit format xxxx | |
cc_cvv | string | Card Security code | |
gateway_id | integer | IncreaseBilling gateway ID | |
gateway_group_id | integer | IncreaseBilling gateway GROUP ID to process transaction, you can configure gateway groups in the member area |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->vaultCreate();
cURL
curl --location --request POST 'https://API_URL/api/vault-add' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'fname=""' \ --form 'lname=""' \ --form 'email=""' \ --form 'address1=""' \ --form 'city=""' \ --form 'state=""' \ --form 'country="US"' \ --form 'postal_code=""' \ --form 'shipping=""' \ --form 'cc_number=""' \ --form 'cc_mo=""' \ --form 'cc_yr=""' \ --form 'cc_ccv=""' \ --form 'gateway_id=""' \
Response
{ "success": 1, "message": "Customer Added", "meta": { "vault_id": "x" }, "transaction": { "user_id": 1, "customer_id": x, "gateway_id": x, "mid_id": null, "total": null, "price": null, "tax": null, "shipping": null, "response": "1", "response_code": "100", "response_text": "Customer Added", "avsresponse": "", "cvvresponse": "", "order_id": "1219991680279618", "transaction_id": "", "type": "vault_add", "vault_id": "x", "checkaba": null, "checkaccount": null, "authcode": "", "tracking_campaigns_id": null, "sandbox": null, "ai_used": null, "ip_address": "x", "bin_list_id": 83983, "gateway_type": "x", "subscription_id": null, "cc_number": null, "first_six": "411111", "last_four": "1111", "card_type": "VISA", "id": 122296 } }
Recurring AI
POST /api/vault-ai
Use AI to maximize a recurring billing transaction. If you have a recurring charge failure, we'll use our custom algorithm to find the optimal retry time, minimizing your attempts and maximizing your rebill success.
IF the charge fails, we'll return a retry date in the meta field along with a retry_reference_id, to process the charge with the AI on the retry date, pass the retry_reference_id along with the request at the specified date. The Response example shows a DECLINE and resulting meta property.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
amount | double | Total Amount of the charge in format x.xx | |
vault_id | integer | IncreaseBilling customer vault ID | |
gateway_id | integer | * | Only required when using a vault_id created in a Gateway (not required for a card_add token ending with "-internal") for billing to a specific Increase Billing gateway ID |
description | string | Order description, product description or any associated description of the transaction | |
retry_reference_id | string | * | Only required when saving a decline, pass this back on the specified retry date in meta->retry_date |
product_id | string | Product ID field for tracking transactions to a number based product ID. (50 character max) | |
product_name | string | Product Name field for tracking transactions to a string (100 character max). | |
product_category | string | Product Category field for tracking transactions to a string (100 character max). | |
3DS | Add 3DS authentication to your transactions, check documentation on left-side menu. |
PHP Client
$sm = new IncreaseBilling(); $sm->order([REQUIRED FIELDS]); $result = $sm->vaultInsight();
cURL
curl --location --request POST 'https://API_URL/api/vault-ai' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'gateway_id="1"' \ --form 'amount=""' \ --form 'vault_id=""' \ --form 'description=""'
Response
{ "success": 1, "message": "DECLINE", "meta": { "retry_date": "2022-06-16 17:27:00", "retry_count": 1, "retry_reference_id": 1655147725, "retry_status": 1, "retry_message": "transaction was declined by processor" }, "transaction": { "user_id": 1, "customer_id": 1, "gateway_id": 3, "mid_id": null, "total": "1.50", "price": "1.50", "tax": null, "shipping": null, "response": "2", "response_code": "220", "response_text": "DECLINE", "avsresponse": "N", "cvvresponse": "", "order_id": "", "transaction_id": "7320800176", "type": "vault_charge", "cc_number": "4xxxxxxxxxxx1111", "vault_id": "", "checkaba": "", "checkaccount": "", "authcode": "123456", "tracking_campaigns_id": null, "sandbox": 1, "ai_used": null, "ip_address": "147.219.60.240", "bin_list_id": null, "gateway_type": "nmi", "subscription_id": null, "first_six": null, "last_four": null, "updated_at": "2022-06-13T19:11:08.000000Z", "created_at": "2022-06-13T19:11:08.000000Z", "id": 258109 }, "customer": { "id": 1, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-06-08T22:40:17.000000Z", "updated_at": "2022-06-13T16:59:09.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288", "vaults": [ { "id": 13017, "user_id": 1, "customer_id": 1, "vault_id": "", "gateway_id": 3, "processor_id": null, "billed": 4, "bin_list_id": 83983, "created_at": "2022-02-10T23:33:41.000000Z", "updated_at": "2022-02-11T03:42:05.000000Z", "card_updated_date": null } ] } }
Update a Recurring Customer Card
POST /api/vault-update
Update a customer payment method with new card information.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
phone | string | Phone # of the customer | |
cc_number | string | Card Number composed of 8 to 19 digits depending on the issuer | |
cc_mo | string | Card Expiration month 01 to 12 | |
cc_yr | string | Card Expiration year in the 4-digit format xxxx | |
cc_cvv | string | Card Security code | |
gateway_id | integer | IncreaseBilling gateway ID | |
vault_id | integer | IncreaseBilling customer vault ID |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->vaultUpdate();
cURL
curl --location --request POST 'https://API_URL/api/vault-update' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'fname=""' \ --form 'lname=""' \ --form 'email=""' \ --form 'address1=""' \ --form 'city=""' \ --form 'state=""' \ --form 'country="US"' \ --form 'postal_code=""' \ --form 'shipping=""' \ --form 'cc_number=""' \ --form 'cc_mo=""' \ --form 'cc_yr=""' \ --form 'cc_ccv=""' \ --form 'gateway_id=""' \ --form 'vault_id=""' \
Response
{ "success": 1, "message": "Customer Updated", "meta": { "vault_id": "x" }, "transaction": { "user_id": 1, "customer_id": x, "gateway_id": x, "mid_id": null, "total": null, "price": null, "tax": null, "shipping": null, "response": "1", "response_code": "100", "response_text": "Customer Added", "avsresponse": "", "cvvresponse": "", "order_id": "1219991680279618", "transaction_id": "", "type": "vault_add", "vault_id": "x", "checkaba": null, "checkaccount": null, "authcode": "", "tracking_campaigns_id": null, "sandbox": null, "ai_used": null, "ip_address": "x", "bin_list_id": 83983, "gateway_type": "x", "subscription_id": null, "cc_number": null, "first_six": "411111", "last_four": "1111", "card_type": "VISA", "id": 122296 } }
Migrate Recurring Customer To A Different Gateway
POST /api/vault-migrate
Move a "Vault" recurring customer to a different gateway. Step 1 Validates the card, if successful, setup tokenized card in the new gateway.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
phone | string | Phone # of the customer | |
cc_number | string | Card Number composed of 8 to 19 digits depending on the issuer | |
cc_mo | string | Card Expiration month 01 to 12 | |
cc_yr | string | Card Expiration year in the 4-digit format xxxx | |
cc_cvv | string | Card Security code | |
gateway_id | integer | IncreaseBilling gateway ID | |
vault_id | integer | IncreaseBilling customer vault ID |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->vaultMigrate();
cURL
curl --location --request POST 'https://API_URL/api/vault-migrate' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'fname=""' \ --form 'lname=""' \ --form 'email=""' \ --form 'address1=""' \ --form 'city=""' \ --form 'state=""' \ --form 'country="US"' \ --form 'postal_code=""' \ --form 'shipping=""' \ --form 'cc_number=""' \ --form 'cc_mo=""' \ --form 'cc_yr=""' \ --form 'cc_ccv=""' \ --form 'gateway_id=""' \ --form 'vault_id=""' \
Response
{ "success": 1, "message": "Customer Updated", "meta": { "vault_id": "x" }, "transaction": { "user_id": 1, "customer_id": x, "gateway_id": x, "mid_id": null, "total": null, "price": null, "tax": null, "shipping": null, "response": "1", "response_code": "100", "response_text": "Customer Added", "avsresponse": "", "cvvresponse": "", "order_id": "1219991680279618", "transaction_id": "", "type": "vault_add", "vault_id": "x", "checkaba": null, "checkaccount": null, "authcode": "", "tracking_campaigns_id": null, "sandbox": null, "ai_used": null, "ip_address": "x", "bin_list_id": 83983, "gateway_type": "x", "subscription_id": null, "cc_number": null, "first_six": "411111", "last_four": "1111", "card_type": "VISA", "id": 122296 } }
Requires Action
POST /api/requires-action-confirmation
Confirm the results of 3DS by posting the result of the Javascript requiredAction callback to Increase Billing, which produces a standard transaction /sale response
If the transaction is declined, handle the results in the same manner you would a standard non-3DS transaction using the results of this request.
These paramters come from the result of the requiredAction() callback. See example.
Attribute | Type | Required | Description |
---|---|---|---|
transaction_id | string | * | Transaction ID from the results of the Javascript requiredAction(jsonMetaProperty, callbackFunction) callback |
gateway_id | string | * | Gateway ID from the results of the Javascript requiredAction(jsonMetaProperty, callbackFunction) callback |
vault_id | string | Vault ID from the results of the Javascript requiredAction(jsonMetaProperty, callbackFunction) callback |
cURL
curl --location --request POST 'https://API_URL/api/requires-action-confirmation' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'gateway_id=""' \ --form 'transaction_id=""' \ --form 'vault_id=""' \
Response
{ "success": 1, "message": "SUCCESS", "meta": [], "transaction": { "user_id": 1, "customer_id": 4, "gateway_id": 58, "mid_id": null, "total": "50.10", "price": "50.00", "tax": ".05", "shipping": ".05", "response": "1", "response_code": "100", "response_text": "SUCCESS", "avsresponse": "", "cvvresponse": "M", "order_id": "141655137213", "transaction_id": "7320273409", "type": "sale", "cc_number": "4xxxxxxxxxxx1111", "vault_id": "", "checkaba": "", "checkaccount": "", "authcode": "123456", "tracking_campaigns_id": "5", "sandbox": 1, "ai_used": null, "ip_address": "147.219.60.240", "bin_list_id": null, "gateway_type": "nmi", "subscription_id": null, "first_six": "411111", "last_four": "1111", "updated_at": "2022-06-13T16:20:16.000000Z", "created_at": "2022-06-13T16:20:16.000000Z", "id": 258106 }, "customer": { "id": 4, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-11-05T00:17:04.000000Z", "updated_at": "2022-06-13T15:49:07.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288" } }
Refund
POST /api/refund
Refund to a customer by transaction ID
Attribute | Type | Required | Description |
---|---|---|---|
amount | double | Amount of the product or service to refund in format x.xx | |
gateway_id | integer | IncreaseBilling gateway ID to process refund | |
transaction_id | integer | IncreaseBilling original transaction ID to refund |
PHP Client
$sm = new IncreaseBilling(); $sm->order([REQUIRED FIELDS]); $result = $sm->refund();
cURL
curl --location --request POST 'https://API_URL/api/refund' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'transaction_id=""' \ --form 'gateway_id=""' \ --form 'amount=""'
Response
{ "success": 1, "message": "SUCCESS", "meta": [], "transaction": { "user_id": 1, "customer_id": 1, "gateway_id": 3, "gateway_type": "nmi", "mid_id": null, "sandbox": 1, "order_id": "", "ai_used": null, "tracking_campaigns_id": null, "product_name": null, "type": "refund", "total": "1.50", "price": null, "shipping": null, "tax": null, "response": 1, "response_code": 100, "response_text": "SUCCESS", "authcode": 123456, "transaction_id": "7321149525", "avsresponse": "N", "cvvresponse": "", "checkaccount": "", "vault_id": "865723865", "checkaba": "", "cc_number": "4xxxxxxxxxxx1111", "first_six": null, "last_four": null, "bin_list_id": null, "ip_address": "147.219.60.240", "linked_transactions_id": 258112, "subscription_id": null, "updated_at": "2022-06-13T21:06:42.000000Z", "created_at": "2022-06-13T21:06:42.000000Z", "id": 258113 }, "customer": { "id": 1, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-06-08T22:40:17.000000Z", "updated_at": "2022-06-13T16:59:09.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288" } }
Validate Card
This action is used for doing a "Verification" on the cardholder's credit card without actually doing an authorization.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
phone | string | Phone # of the customer | |
cc_number | string | Card Number composed of 8 to 19 digits depending on the issuer | |
cc_mo | string | Card Expiration month 01 to 12 | |
cc_yr | string | Card Expiration year in the 4-digit format xxxx | |
cc_cvv | string | Card Security code | |
gateway_id | integer | IncreaseBilling gateway ID to process transaction | |
gateway_group_id | integer | IncreaseBilling gateway GROUP ID to process transaction, you can configure gateway groups in the member area | |
vault_add | integer | Send "1" to create a vault_id if the transaction is successful, results in response->meta->vault_id, make sure to save response->meta->gateway_id for future charges. | |
card_add | integer | Send "1" to create a tokenized card which can be used for future billing with a Recurring request, results in response->meta->vault_id. |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->validate();
cURL
curl --location --request POST 'https://API_URL/api/validate' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'fname=""' \ --form 'lname=""' \ --form 'email=""' \ --form 'address1=""' \ --form 'city=""' \ --form 'state=""' \ --form 'country="US"' \ --form 'postal_code=""' \ --form 'shipping=""' \ --form 'cc_number=""' \ --form 'cc_mo=""' \ --form 'cc_yr=""' \ --form 'cc_ccv=""' \
Response
{ "success": 1, "message": "SUCCESS", "meta": [], "transaction": { "user_id": 1, "customer_id": 4, "gateway_id": 3, "mid_id": null, "total": "0.00", "price": null, "tax": null, "shipping": null, "response": "1", "response_code": "100", "response_text": "SUCCESS", "avsresponse": "", "cvvresponse": "M", "order_id": "", "transaction_id": "7320168307", "type": "validate", "cc_number": "4xxxxxxxxxxx1111", "vault_id": "", "checkaba": "", "checkaccount": "", "authcode": "", "tracking_campaigns_id": null, "sandbox": 1, "ai_used": null, "ip_address": "147.219.60.240", "bin_list_id": null, "gateway_type": "nmi", "subscription_id": null, "first_six": "411111", "last_four": "1111", "updated_at": "2022-06-13T15:49:09.000000Z", "created_at": "2022-06-13T15:49:09.000000Z", "id": 258104 }, "customer": { "id": 4, "user_id": 1, "email": "", "fname": "", "lname": "", "address1": "", "address2": null, "city": "Fitchburg", "state": "WI", "country": "US", "postal_code": "53711", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-11-05T00:17:04.000000Z", "updated_at": "2022-02-09T00:38:56.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288" } }
Void
POST /api/void
Void a transaction ID, returning the entire total to the customer and cancelling the original transaction
Attribute | Type | Required | Description |
---|---|---|---|
gateway_id | integer | IncreaseBilling gateway ID to process transaction | |
transaction_id | integer | IncreaseBilling transaction ID |
PHP Client
$sm = new IncreaseBilling(); $sm->order([REQUIRED FIELDS]); $result = $sm->void();
cURL
curl --location --request POST 'https://API_URL/api/void' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \ --form 'transaction_id=""' \ --form 'gateway_id=""' \
Response
{ "success": true, "transaction": { "response": "1", "responsetext": "Transaction Void Successful", "authcode": "123456", "transactionid": "6600447294", "avsresponse": "", "cvvresponse": "", "orderid": "131632258748", "type": "void", "response_code": "100", "cc_number": "4xxxxxxxxxxx1111", "customer_vault_id": "", "checkaba": "", "checkaccount": "" }, "message": "", "gateway": 1, "mid": false }
Create a Subscription Model
POST /api/subscription-create
Add a new subscription model
Attribute | Type | Required | Description |
---|---|---|---|
name | string | * | Name of subscription |
amount | integer | * | Amount to bill |
active | integer | * | Enable or disable subscription model for all subscribers |
day_frequency | integer | How often in DAYS to rebill subscribers, required if month_frequency is empty | |
month_frequency | integer | How often in MONTHS to rebill subscribers, required if day_frequency is empty | |
days_before_first_bill | integer | Number of days from datetime of subscribing to process first bill for a subscriber | |
webhook | string | URL to send subscription transaction results to |
cURL
curl --location --request POST 'https://API_URL/api/subscription-create' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'name="Testing"' \ --form 'amount="10.00"' \ --form 'active="1"' \ --form 'day_frequency="30"' \ --form 'days_before_first_bill="10"' \ --form 'webhook="http://example.com"'
Response
{ { "success": 1, "message": "subscription created", "subscription": { "name": "Testing", "amount": "10.00", "day_frequency": "30", "month_frequency": null, "days_before_first_bill": "10", "days_before_retry": 3, "max_payments": 999, "webhook": "http://example.com", "active": "1", "updated_at": "2024-02-08T14:59:09.000000Z", "created_at": "2024-02-08T14:59:09.000000Z", "id": 7 } } }
Update a Subscription Model
POST /api/subscription-update
Update an existing subscription model
Attribute | Type | Required | Description |
---|---|---|---|
subscription_id | integer | ID of subscription in Increase Billing | |
name | string | Name of subscription | |
amount | integer | Amount to bill | |
active | integer | Enable or disable subscription model for all subscribers | |
day_frequency | integer | How often in DAYS to rebill subscribers, required if month_frequency is empty | |
month_frequency | integer | How often in MONTHS to rebill subscribers, required if day_frequency is empty | |
days_before_first_bill | integer | Number of days from datetime of subscribing to process first bill for a subscriber | |
webhook | string | URL to send subscription transaction results to |
cURL
curl --location --request POST 'https://API_URL/api/subscription-update' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'subscription_id="1"' \ --form 'name="Testing"' \ --form 'amount="10.00"' \ --form 'active="1"' \ --form 'day_frequency="30"' \ --form 'days_before_first_bill="10"' \ --form 'webhook="http://example.com"'
Response
{ { "success": 1, "message": "subscription updated", "subscription": { "name": "Testing", "amount": "10.00", "day_frequency": "30", "month_frequency": null, "days_before_first_bill": "10", "days_before_retry": 3, "max_payments": 999, "webhook": "http://example.com", "active": "1", "updated_at": "2024-02-08T14:59:09.000000Z", "created_at": "2024-02-08T14:59:09.000000Z", "id": 1 } } }
Get a Subscription Model
POST /api/subscription-get
Get a subscription model by subscription ID
Attribute | Type | Required | Description |
---|---|---|---|
subscription_id | integer | ID of subscription in Increase Billing |
cURL
curl --location --request GET 'https://API_URL/api/subscription-get' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'subscription_id=1' \
Response
{ { "success": 1, "message": "", "subscriptions": [ { "id": 1, "name": "Testing", "amount": "10.00", "day_frequency": 30, "month_frequency": 0, "days_before_first_bill": 10, "days_before_retry": 3, "max_payments": 999, "descriptor": null, "active": 1, "webhook": "http://warmplasma.com", "active_customers": 0, "inactive_customers": 0, "billed": 0, "created_at": "2023-01-20T03:53:10.000000Z", "updated_at": "2024-02-08T15:37:49.000000Z", "prefer_gateway_id": null }, ] } }
Add an existing customer to an existing subscription model
POST /api/subscriber-add
Process a sale charge for a customer stored in the vault.
Attribute | Type | Required | Description |
---|---|---|---|
customer_id | integer | IncreaseBilling Customer ID of the customer to create a subscription for | |
subscription_id | integer | IncreaseBilling Subscription ID to add the customer to |
PHP Client
$sm = new IncreaseBilling(); $sm->order([REQUIRED FIELDS]); $result = $sm->subscriberAdd();
cURL
curl --location --request POST 'https://API_URL/api/subscriber-add' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'customer_id=""' \ --form 'subscription_id=""'
Response
{ "success": 1, "message": "Subscription created!", "meta": { "customer_id": 4, "subscription_id": 7, "active": 1, "next_bill": "2022-08-24 12:14:57", "updated_at": "2022-07-25T17:14:57.000000Z", "created_at": "2022-07-25T17:14:57.000000Z", "id": 16 }, "transaction": { "user_id": 1, "customer_id": 1, "gateway_id": 3, "mid_id": null, "total": "1.50", "price": "1.50", "tax": null, "shipping": null, "response": "1", "response_code": "100", "response_text": "SUCCESS", "avsresponse": "N", "cvvresponse": "", "order_id": "", "transaction_id": "7321143756", "type": "vault_charge", "cc_number": "4xxxxxxxxxxx1111", "vault_id": "865723865", "checkaba": "", "checkaccount": "", "authcode": "123456", "tracking_campaigns_id": null, "sandbox": 1, "ai_used": null, "ip_address": "147.219.60.240", "bin_list_id": null, "gateway_type": "nmi", "subscription_id": null, "first_six": null, "last_four": null, "updated_at": "2022-06-13T21:04:38.000000Z", "created_at": "2022-06-13T21:04:38.000000Z", "id": 258112 }, "customer": { "id": 1, "user_id": 1, "email": "atesting15@thing.com", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-06-08T22:40:17.000000Z", "updated_at": "2022-06-13T16:59:09.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288", "vault": [ { "id": 1, "user_id": 1, "customer_id": 1, "vault_id": "1052984295", "gateway_id": 3, "processor_id": null, "billed": 0, "bin_list_id": null, "created_at": "2021-06-17T20:20:48.000000Z", "updated_at": "2022-01-03T21:56:19.000000Z", "card_updated_date": null } ] } }
Create a Recurring Customer and Add them to a Subscription ID
POST /api/subscriber-create
Add a customer to the "vault" along with their card information, then add them to a subscription model.
Attribute | Type | Required | Description |
---|---|---|---|
string | * | Email Address of the customer (alternatively use customer_identifier) | |
customer_identifier | string | * | Unique customer identifier (alternatively use email) |
fname | string | First Name of the customer | |
lname | string | Last Name of the customer | |
address1 | string | Street Address of the customer | |
address2 | string | Secondary Address of the customer e.g. Apt. #, P.O. Box | |
city | string | City of Residence of the customer | |
state | string | State of Residence of the customer | |
country | string | Country of Residence of the customer | |
postal_code | string | Postal Code of the customer | |
phone | string | Phone # of the customer | |
cc_number | string | Card Number composed of 8 to 19 digits depending on the issuer | |
cc_mo | string | Card Expiration month 01 to 12 | |
cc_yr | string | Card Expiration year in the 4-digit format xxxx | |
cc_cvv | string | Card Security code | |
gateway_id | integer | IncreaseBilling gateway ID | |
gateway_group_id | integer | IncreaseBilling gateway GROUP ID to process transaction, you can configure gateway groups in the member area | |
subscription_id | integer | IncreaseBilling Subscription ID to add the customer to |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $sm->order([REQUIRED FIELDS]); $result = $sm->subscriberCreate();
cURL
curl --location --request POST 'https://API_URL/api/subscriber-create' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'fname=""' \ --form 'lname=""' \ --form 'email=""' \ --form 'address1=""' \ --form 'city=""' \ --form 'state=""' \ --form 'country="US"' \ --form 'postal_code=""' \ --form 'shipping=""' \ --form 'cc_number=""' \ --form 'cc_mo=""' \ --form 'cc_yr=""' \ --form 'cc_ccv=""' \ --form 'gateway_id=""' \ --form 'subscription_id=""' \
Response
{ "success": 1, "message": "Subscription created!", "meta": { "customer_id": 1, "subscription_id": 5, "active": 1, "next_bill": "2022-08-24 13:14:32", "updated_at": "2022-07-25T18:14:32.000000Z", "created_at": "2022-07-25T18:14:32.000000Z", "id": 17 }, "transaction": { "user_id": 1, "customer_id": 1, "gateway_id": 3, "mid_id": null, "total": "0.00", "price": null, "tax": null, "shipping": null, "response": "1", "response_code": "100", "response_text": "Customer Added", "avsresponse": "", "cvvresponse": "", "order_id": "", "transaction_id": "", "type": "vault_add", "cc_number": "4xxxxxxxxxxx1111", "vault_id": "570982526", "checkaba": "", "checkaccount": "", "authcode": "", "tracking_campaigns_id": null, "sandbox": 1, "ai_used": null, "ip_address": "147.219.60.240", "bin_list_id": null, "gateway_type": "nmi", "subscription_id": "5", "first_six": "411111", "last_four": "1111", "updated_at": "2022-07-25T18:14:31.000000Z", "created_at": "2022-07-25T18:14:31.000000Z", "id": 258191 }, "customer": { "id": 1, "user_id": 1, "email": "haberman13@gmail.com", "fname": "Eric", "lname": "Haberman", "address1": "5507 Quarry Hill Dr", "address2": null, "city": "Madison", "state": "WI", "country": "US", "postal_code": "53711", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-06-08T22:40:17.000000Z", "updated_at": "2022-07-25T18:14:31.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288", "vault": [ { "id": 1, "user_id": 1, "customer_id": 1, "vault_id": "1052984295", "gateway_id": 1, "processor_id": null, "billed": 0, "bin_list_id": null, "created_at": "2021-06-17T20:20:48.000000Z", "updated_at": "2022-01-03T21:56:19.000000Z", "card_updated_date": null }, ] } }
Get subscriptions for an IncreaseBilling customer ID
POST /api/subscriber-get
Get subscription information for a customer ID in the IncreaseBilling system.
Attribute | Type | Required | Description |
---|---|---|---|
customer_id | integer | IncreaseBilling Customer ID of the customer to create a subscription for |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $result = $sm->subscriberGet();
cURL
curl --location --request POST 'https://API_URL/api/subscriber-get' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'customer_id=""' \
Response
{ "success": 1, "message": "See meta property", "meta": [ { "id": 1, "customer_id": 4, "customers_vault_id": null, "subscription_id": 5, "active": 1, "billed": 12, "last_billed": "2022-07-01 15:40:06", "next_bill": "2022-07-31 15:40:02", "cancel_date": null, "ai_enabled": 0, "attempts": 0, "retry_reference_id": null, "created_at": "2022-05-01T04:44:44.000000Z", "updated_at": "2022-07-01T20:40:06.000000Z", "last_transaction_id": 258125 }, { "id": 16, "customer_id": 4, "customers_vault_id": null, "subscription_id": 7, "active": 1, "billed": 0, "last_billed": null, "next_bill": "2022-08-24 12:14:57", "cancel_date": null, "ai_enabled": 0, "attempts": 0, "retry_reference_id": null, "created_at": "2022-07-25T17:14:57.000000Z", "updated_at": "2022-07-25T17:14:57.000000Z", "last_transaction_id": null } ], "transaction": null, "customer": { "id": 1, "user_id": 1, "email": "atesting15@thing.com", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-06-08T22:40:17.000000Z", "updated_at": "2022-06-13T16:59:09.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288", "vault": [ { "id": 1, "user_id": 1, "customer_id": 1, "vault_id": "1052984295", "gateway_id": 3, "processor_id": null, "billed": 0, "bin_list_id": null, "created_at": "2021-06-17T20:20:48.000000Z", "updated_at": "2022-01-03T21:56:19.000000Z", "card_updated_date": null } ] } }
Disable an IncreaseBilling subscription for a customer ID and subscription ID
POST /api/subscriber-disable
Disable subscription for a customer ID and subscription ID.
Attribute | Type | Required | Description |
---|---|---|---|
customer_id | integer | IncreaseBilling Customer ID of the customer to disable the subscription for | |
subscription_id | integer | IncreaseBilling Subscription ID |
PHP Client
$sm = new IncreaseBilling(); $sm->customer([REQUIRED FIELDS]); $result = $sm->subscriberDisable();
cURL
curl --location --request POST 'https://API_URL/api/subscriber-disable' --header 'Accept: application/json' --header 'Authorization: Bearer API_KEY' --form 'customer_id=""' \ --form 'subscription_id=""' \
Response
{ "success": 1, "message": "Subscription(s) disabled", "meta": [ [ { "id": 16, "customer_id": 4, "customers_vault_id": null, "subscription_id": 7, "active": 0, "billed": 0, "last_billed": null, "next_bill": "2022-08-24 12:14:57", "cancel_date": null, "ai_enabled": 0, "attempts": 0, "retry_reference_id": null, "created_at": "2022-07-25T17:14:57.000000Z", "updated_at": "2022-07-25T18:33:08.000000Z", "last_transaction_id": null } ] ], "transaction": null, "customer": { "id": 1, "user_id": 1, "email": "atesting15@thing.com", "fname": "", "lname": "", "address1": "", "address2": null, "city": "", "state": "", "country": "", "postal_code": "", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2021-06-08T22:40:17.000000Z", "updated_at": "2022-06-13T16:59:09.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": "28288", "vault": [ { "id": 1, "user_id": 1, "customer_id": 1, "vault_id": "1052984295", "gateway_id": 3, "processor_id": null, "billed": 0, "bin_list_id": null, "created_at": "2021-06-17T20:20:48.000000Z", "updated_at": "2022-01-03T21:56:19.000000Z", "card_updated_date": null } ] } }
Get Customer Card Update Link
POST /api/get-card-update-link
Generate a link for a customer to update their stored card info
Attribute | Type | Required | Description |
---|---|---|---|
string | Customer email | ||
gateway_id | integer | Increase Billing gateway ID | |
vault_id | string | Vault ID associated with the customer and gateway_id |
cURL
curl --location --request POST 'https://API_URL/api/get-card-update-link' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \
Response
{ "success": 1, "message": "Link generated", "link": "IFRAME_URL/?k=FRxQJQtSMmGRocAZOB8ei1BUmPw9WIbCjKNhSSKN&t=", "key": "FRxQJQtSMmGRocAZOB8ei1BUmPw9WIbCjKNhSSKN" }
Get Customer Information
GET /api/get-customers
Results are limited to 200 per request, use offset to move the cursor forward. Less than 200 records returned in the 'total' property indicates last in the result set.
Attribute | Type | Required | Description |
---|---|---|---|
string | Customer email | ||
customer_identifier | string | Customer Identifier | |
customer_id | string | Internal customer_id returned in transaction results | |
offset | integer | Move the query cursor forward to offset |
cURL
curl --location --request GET 'https://API_URL/api/get-customers' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \
Response
{ "total": 200, "success": 1, "message": "", "customers": [ { "id": 1, "user_id": 1, "email": "xxxxxx", "fname": "xxxxxx", "lname": "xxxxxx", "address1": "xxxxxx", "address2": null, "city": "xxxxxx", "state": "xxxxxx", "country": "xxxxxx", "postal_code": "xxxxxx", "phone": "xxxxxx", "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": "xxxxxx", "created_at": "2021-06-08T21:40:17.000000Z", "updated_at": "2024-01-10T21:26:36.000000Z", "stripe": 1, "stripe_id": "xxxxxx", "customer_identifier": "28288", "chargeback": null, "gateway_id": 3, "authorize_id": null }, } }
Get Detailed Transaction Records
GET /api/get-detailed-transactions
Results are limited to 50 per request, use offset to move the cursor forward. Less than 50 records returned in the 'total' property indicates last in the result set.
Attribute | Type | Required | Description |
---|---|---|---|
type | string | IncreaseBilling transaction type - sale, vault_charge, refund, void, chargeback | |
start_date | string | Get transactions created on or after start_date (format YYYY-MM-DD) | |
end_date | string | Get transactions created on or before end_date (format YYYY-MM-DD) | |
offset | integer | Move the query cursor forward to offset |
cURL
curl --location --request GET 'https://API_URL/api/get-detailed-transactions' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \
Response
{ "total": 50, "success": 1, "message": "", "transactions": [ { "total": "13.05", "price": "13.05", "shipping": "0.00", "tax": "0.00", "response": 1, "response_code": 100, "response_text": "SUCCESS", "transaction_id": "8412451513", "order_id": "1220031685976978", "customer_id": 22003, "gateway_id": 3, "gateway_type": "nmi", "type": "sale", "avsresponse": "N", "cvvresponse": "M", "ip_address": "35.148.238.126", "first_six": "411111", "last_four": "1111", "product_id": null, "product_name": null, "retry": 0, "created_at": "2023-06-05T13:56:18.000000Z", "bin": { "id": 83983, "bin": 411111, "brand": "VISA", "type": "CREDIT", "category": "", "issuer": "JPMORGAN CHASE BANK, N.A.", "country": "United States", "created_at": "2020-06-09T14:52:22.000000Z", "updated_at": "2020-06-09T14:52:22.000000Z" }, "owner": { "id": 22003, "user_id": 1, "email": "*", "fname": "*", "lname": "*", "address1": "*", "address2": null, "city": "*", "state": "*", "country": "US", "postal_code": "*", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2023-04-26T13:09:09.000000Z", "updated_at": "2023-06-20T16:37:00.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": null, "chargeback": null, "gateway_id": * } }, ]
Get Gateways
GET /api/get-gateways
Get Gateway information from IncreaseBilling
Attribute | Type | Required | Description |
---|---|---|---|
No Requirements |
PHP Client
$sm = new IncreaseBilling(); $sm->order([REQUIRED FIELDS]); $result = $sm->getGateways();
cURL
curl --location --request GET 'https://API_URL/api/get-gateways' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \
Response
[ { "name": "Name of Gateway", "id": 1, "type": "nmi", "active": 0, "sandbox": null, "cap_current": "0.00", "cap": "100000.00", "cap_percent": "0.00", "decline_percent": "39.97", "stats": { "id": 1405941, "account": "An LLC", "date": "2023-04-12", "gateway_id": 1, "three_ds_initialize": 0, "three_ds_success": 0, "sale": 0, "sale_total": "0.00", "sale_all_time": 1500, "sale_ai": 0, "sale_avg": "62.34", "sale_decline": 0, "sale_decline_all_time": 794, "sale_decline_total": "0.00", "vault_decline": 0, "vault_decline_all_time": 4270, "vault_decline_unique": 1115, "vault_decline_total": "0.00", "vault_charge": 0, "vault_charge_total": "0.00", "vault_charge_all_time": 4079, "vault_add": 0, "vault_ai": 0, "vault_charge_avg": "418.35", "refund": 0, "refund_total": "0.00", "refund_avg": "52.17", "void": 0, "void_total": "0.00", "void_avg": "5.14", "chargeback": 0, "chargeback_total": "0.00", "validate": 0, "created_at": "2023-04-12T16:00:09.000000Z", "updated_at": "2023-04-12T16:00:09.000000Z", "sale_all_time_total": "22566.86", "sale_decline_all_time_total": "12482.86" } ]
Change Gateway's Active Status
POST /api/active-gateway
Activate or deactivate a Gateway in IncreaseBilling
Attribute | Type | Required | Description |
---|---|---|---|
gateway_id | integer | IncreaseBilling gateway ID to process transaction | |
active | integer | Send 1 to activate and 0 to deactivate (not you cannot deactivate the default gateway before selecting another default gateway first). |
cURL
curl --location --request POST 'https://API_URL/api/active-gateway' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \
Response
{ "success": 1, "gateway": { "name": "NMI Kosoweb", "id": 59, "type": "nmi", "active": 1, "sandbox": null, "cap_current": "0.20", "cap": "10000.00", "cap_percent": "0.00", "decline_percent": "25.00", "stats": { "id": 1406076, "account": "Protech LLC", "date": "2023-04-12", "gateway_id": 59, "three_ds_initialize": 0, "three_ds_success": 0, "sale": 0, "sale_total": "0.00", "sale_all_time": 2, "sale_ai": 0, "sale_avg": "0.06", "sale_decline": 1, "sale_decline_all_time": 5, "sale_decline_total": "0.05", "vault_decline": 0, "vault_decline_all_time": 0, "vault_decline_unique": 0, "vault_decline_total": "0.00", "vault_charge": 0, "vault_charge_total": "0.00", "vault_charge_all_time": 0, "vault_add": 0, "vault_ai": 0, "vault_charge_avg": "0.00", "refund": 0, "refund_total": "0.00", "refund_avg": "0.00", "void": 0, "void_total": "0.00", "void_avg": "0.00", "chargeback": 0, "chargeback_total": "0.00", "validate": 1, "created_at": "2023-04-12T17:00:28.000000Z", "updated_at": "2023-04-12T17:00:28.000000Z", "sale_all_time_total": "20.00", "sale_decline_all_time_total": "4.20" } } }
Get Transaction Records
GET /api/get-transactions
Results are limited to 200 per request, use offset to move the cursor forward. Less than 200 records returned in the 'total' property indicates last in the result set.
Attribute | Type | Required | Description |
---|---|---|---|
type | string | IncreaseBilling transaction type - sale, vault_charge, refund, void, chargeback | |
start_date | string | Get transactions created on or after start_date (format YYYY-MM-DD) | |
end_date | string | Get transactions created on or before end_date (format YYYY-MM-DD) | |
order_id | string | Get transactions with order_id | |
transaction_id | string | Get transactions with transaction_id | |
customer_id | string | Get transactions with customer_id | |
total | float | Get transactions with total in format x.xx | |
offset | integer | Move the query cursor forward to offset |
cURL
curl --location --request GET 'https://API_URL/api/get-transactions' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \
Response
{ "total": 200, "success": 1, "message": "", "transactions": [ { "id": 55735, "total": "39.95", "response": 2, "response_code": 200, "response_text": "Decline", "transaction_id": "333", "customer_id": 10407, "gateway_id": 23, "type": "vault_charge", "created_at": "2022-06-02T19:00:44.000000Z" }, .... ] }
Get Detailed Transaction Records
GET /api/get-detailed-transactions
Results are limited to 50 per request, use offset to move the cursor forward. Less than 50 records returned in the 'total' property indicates last in the result set.
Attribute | Type | Required | Description |
---|---|---|---|
type | string | IncreaseBilling transaction type - sale, vault_charge, refund, void, chargeback | |
start_date | string | Get transactions created on or after start_date (format YYYY-MM-DD) | |
end_date | string | Get transactions created on or before end_date (format YYYY-MM-DD) | |
order_id | string | Get transactions with order_id | |
transaction_id | string | Get transactions with transaction_id | |
customer_id | string | Get transactions with customer_id | |
total | float | Get transactions with total in format x.xx | |
offset | integer | Move the query cursor forward to offset |
cURL
curl --location --request GET 'https://API_URL/api/get-detailed-transactions' \ --header 'Accept: application/json' \ --header 'Authorization: Bearer API_KEY' \
Response
{ "total": 50, "success": 1, "message": "", "transactions": [ { "total": "13.05", "price": "13.05", "shipping": "0.00", "tax": "0.00", "response": 1, "response_code": 100, "response_text": "SUCCESS", "transaction_id": "8412451513", "order_id": "1220031685976978", "customer_id": 22003, "gateway_id": 3, "gateway_type": "nmi", "type": "sale", "avsresponse": "N", "cvvresponse": "M", "ip_address": "35.148.238.126", "first_six": "411111", "last_four": "1111", "product_id": null, "product_name": null, "retry": 0, "created_at": "2023-06-05T13:56:18.000000Z", "bin": { "id": 83983, "bin": 411111, "brand": "VISA", "type": "CREDIT", "category": "", "issuer": "JPMORGAN CHASE BANK, N.A.", "country": "United States", "created_at": "2020-06-09T14:52:22.000000Z", "updated_at": "2020-06-09T14:52:22.000000Z" }, "owner": { "id": 22003, "user_id": 1, "email": "*", "fname": "*", "lname": "*", "address1": "*", "address2": null, "city": "*", "state": "*", "country": "US", "postal_code": "*", "phone": null, "shipping_address1": null, "shipping_address2": null, "shipping_city": null, "shipping_state": null, "shipping_country": null, "shipping_postal_code": null, "ip_address": null, "created_at": "2023-04-26T13:09:09.000000Z", "updated_at": "2023-06-20T16:37:00.000000Z", "stripe": null, "stripe_id": null, "customer_identifier": null, "chargeback": null, "gateway_id": * } }, ]